The article shows how to create an Excel drop down that allows users to select multiple items with or without duplicates.
Excel has come a long way since its inception and introduces more and more useful features with each new release. In Excel 365, they've added the ability to search within data validation lists, which is a huge time-saver when working with large sets of data. However, even with this new option, out-of-the-box Excel still only allows selecting one item from a predefined list of options. But fear not, as there is a solution. By using VBA, you can create drop-down lists with multiple selections. With the ability to prevent duplicates and remove incorrect items, this feature can streamline data input and improve accuracy in your Excel spreadsheets.
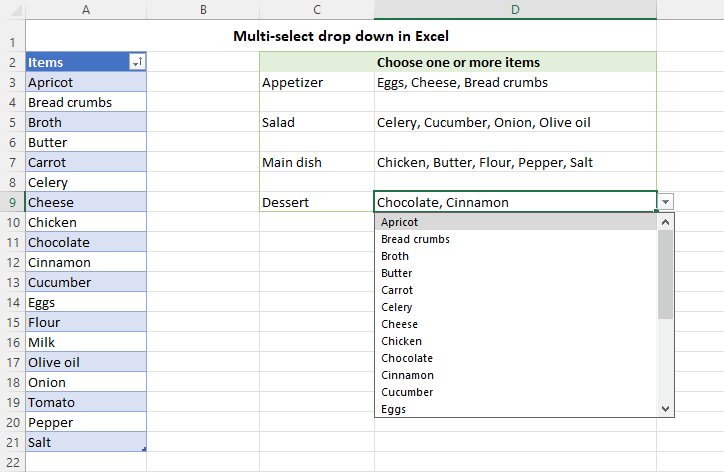
How to make Excel drop down with multiple selections
Creating a multi-select drop down list in Excel is a two-part process:
- First, you make a regular data validation list in one or more cells.
- And then, insert the VBA code at the back end of the target worksheet.
It also works in the reverse order :)
Create a normal drop-down list
To insert a drop down list in Excel, you use the Data Validation feature. The steps slightly vary depending on whether the source items are in a regular range, named range, or an Excel table.
From my experience, the best option is to create a data validation list from a table. As Excel tables are dynamic by nature, a related dropdown will expand or contract automatically as you add or remove items to/from the table.
For this example, we are going to use the table with the plain name Table1, which resides in A2:A25 in the screenshot below. To make a picklist from this table, the steps are:
- Select one or more cells for your dropdown (D3:D7 in our case).
- On the Data tab, in the Data Tools group, click Data Validation.
- In the Allow drop-down box, select List.
- In the Source box, enter the formula that indirectly refers to Table1's column named Items.
=INDIRECT("Table1[Items]")
- When done, click OK.
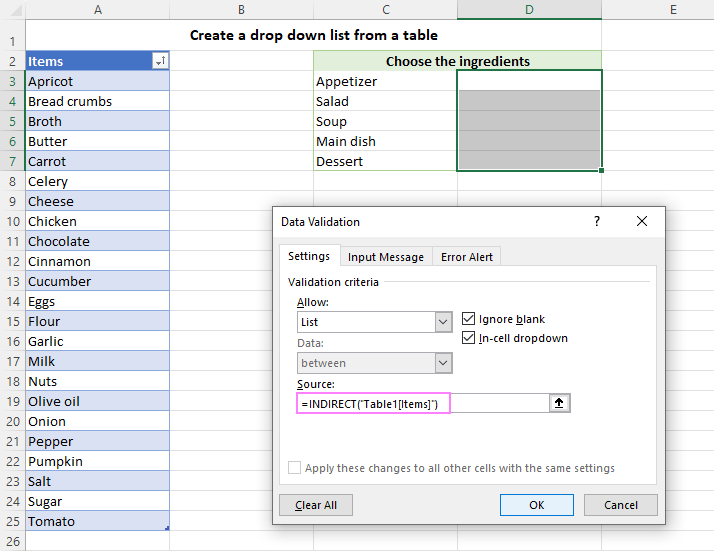
The result will be an expandable and automatically updatable drop-down list that only allows selecting one item.
Tip. If the method described above is not suitable for you for some reason, you can create a dropdown from a regular range or named range. The detailed instructions are here: How to create Excel data validation list.
Insert VBA code to allow multiple selections
This is the core part of the process that does the magic. To turn a regular single-selection picklist into a multi-select dropdown, you need to insert one of these codes in the back end of your target worksheet:
- VBA code for multi-select drop down with duplicates
- VBA code for multi-select drop down without duplicates
- VBA code for multi-selection dropdown with item removal
To add VBA code to your worksheet, follow these steps:
- Open the Visual Basic Editor by pressing Alt + F11 or clicking the Developer tab > Visual Basic. If you don't have this tab on your Excel ribbon, see how to add Developer tab.
- In the Project Explorer pane at the left, double-click on the name of the worksheet that contains your drop-down list. This will open the Code window for that sheet.
Or you can right-click the sheet's tab and choose View Code from the context menu. This will open the Code window for a given sheet straight away.
- In the Code window, paste the VBA code.
- Close the VB Editor and save your file as a Macro-Enabled Workbook (.xlsm).
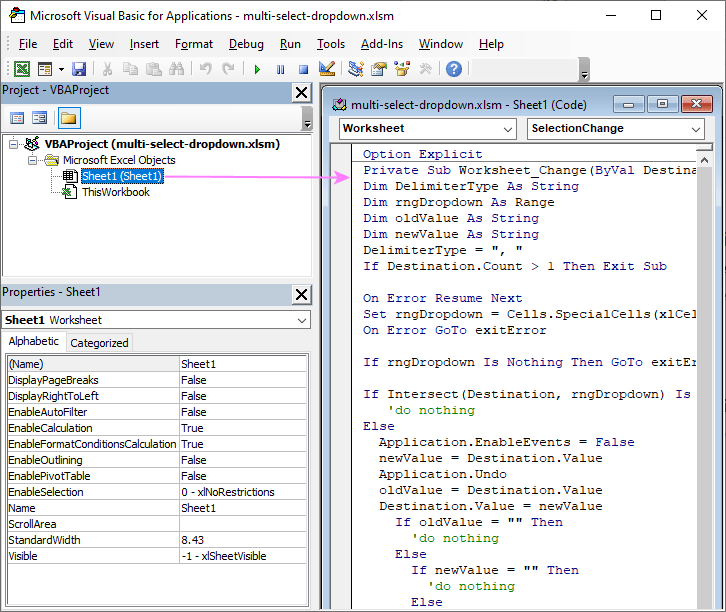
That's it! When you go back to the worksheet, your drop-down list will allow you to select multiple items:
VBA code to select multiple items in dropdown list
Below is the code to make a data validation list that allows selecting multiple items, including repeated selections:
How this code works:
- The code enables multiple selections in all drop down lists on a particular sheet. You do not need to specify the target cell or range reference in the code.
- The code is worksheet specific, so be sure to add it to each sheet where you want to allow multiple selections in drop down lists.
- This code allows repetition, i.e. selecting the same item several times.
- The selected items are separated with a comma and a space. To change the delimiter, replace ", " with the character you want in DelimiterType = ", " (line 7 in the code above).
Note. The same character cannot be used as both the delimiter and part of the dropdown items. In our code, the default delimiter is a comma followed by a space (", "), so this combination of characters should not appear anywhere within the dropdown items themselves to avoid conflicts. If you change the delimiter to a single space (" "), bear in mind that the code will only function correctly for single-word items, failing to handle multi-word items containing spaces.
Excel multi-select dropdown without duplicates
When selecting from a large list, users can sometimes pick the same item more than once without noticing. The code below solves the problem of duplicates in a multi-selection data validation drop down list. It lets users pick a particular item only once. If you try to select the same item again, nothing will happen. Pretty cool, right?
Multi-selection dropdown with item removal
When users need to select multiple options but can make mistakes or change their minds during the selection process, a multi selection dropdown that allows for the removal of incorrect items can be a lifesaver.
Consider a scenario where you need to assign multiple tasks to team members using a drop-down list. With Excel's default functionality, the only way to remove an incorrectly assigned task is by clearing the entire contents of the cell and starting over. With the ability to remove individual items from the selection, the team can effortlessly modify task assignments without confusion or errors.
The code below implements the item removal functionality in a simple and intuitive way: the first click on an item adds it to the selection, and a second click on the same item removes it from the selection.
The below demo highlights how the multi selection dropdown with removal functionality works in Excel. The users can select multiple options from the data validation list and make adjustments on the fly. A streamlined and effective approach to managing selections!
How to make a multiple selection dropdown with custom delimiter
The character that separates items in the selection is set in the DelimiterType parameter. In all the codes, the default value of this parameter is ", " (a comma and a space) and it is located in line 7. To use a different separator, you can replace ", " with the desired character. For instance:
- To separate the selected items with a space, use DelimiterType = " ".
- To separate with a semicolon, use DelimiterType = "; " or DelimiterType = ";" (with or without a space, respectively).
- To separate with a vertical bar, use DelimiterType = " | ".
For example, if you change the delimiter to a vertical slash, your multi-select picklist will look as follows:
How to create dropdown with multiple selections in separate lines
To get each selection in a separate line in the same cell, set DelimiterType to Vbcrlf. In VBA, it is a constant for the carriage return and line feed characters.
More precisely, you change this code line:
DelimiterType = ","
to this one:
DelimiterType = vbCrLf
As a result, each item that you select from the dropdown list will appear in a new line:
How to create multi-select dropdown for specific columns, rows, cells and ranges
All the codes described in this tutorial work across an entire sheet. However, you can easily modify any of the codes, so it only applies to specific cells, rows, or columns as needed. For this, find this line of code:
If rngDropdown Is Nothing Then GoTo exitError
Add immediately after it, add a new line specifying where to allow multiple selections, as explained in the below examples.
Multi-select drop-down for specific columns
To allow selecting multiple items in a certain column, add this code:
If Not Destination.Column = 4 Then GoTo exitError
Where "4" is the number of the target column. In this case, the multi-select dropdown will be only enabled in column D. In all other columns, dropdown lists will be limited to a single selection.
To target several columns, specify their numbers using this code:
If Destination.Column <> 4 And Destination.Column <> 6 Then GoTo exitError
In this case, the multi-select dropdown will be available in columns D (4) and F (6).
Multi-selection dropdown for certain rows
To insert multiple drop-downs in a specific row, use this code:
If Not Destination.Row = 3 Then GoTo exitError
In this example, replace "3" with the row number where you want to enable multi-select dropdowns.
To target multiple rows, the code is as follows:
If Destination.Row <> 3 And Destination.Row <> 5 Then GoTo exitError
Where "3" and "5" are the rows where selecting multiple items is allowed.
Multiple selections in specific cells
To enable multiple selections in particular cells, add one of the below code lines.
For a single cell:
If Not Destination.Address = "$D$3" Then GoTo exitError
For multiple cells:
If Destination.Address <> "$D$3" And Destination.Address <> "$F$6" Then GoTo exitError
Just remember to replace "$D$3" and "$F$6" with the addresses of your target cells.
Multi-select drop-down for specific range
To limit your multi-select dropdown to a particular range, replace this line of code:
If Intersect(Destination, rngDropdown) Is Nothing Then
with this one:
If Intersect(ActiveCell, Range("C3:D10")) Is Nothing Or Intersect(Destination, rngDropdown) Is Nothing Then
The range of interest is specified directly in the code (C3:D10 in the above example). This modification offers a more efficient approach to handing ranges - instead of individually listing 16 cells, you use a single range reference.
How to enable multi-selection functionality in protected sheet
To enable a multi-select dropdown functionality in a protected worksheet, simply insert the following code into the sheet where you've added the primary code.
Before adding this code to your worksheet, remember to replace "password" with the actual password you used to protect the sheet. And this is the only change that needs to be made. The code will automatically detect the presence of a dropdown list in a given cell and allow editing of that cell. In all other cells, editing will remain restricted.
Note. Please be aware that including your real password in the code could lead to a security risk. To ensure the safety of your workbook, store it in a secure location that is protected against unauthorized access or use.
So, there you have it - an Excel dropdown list with multiple selections. Adding this awesome feature to your spreadsheets will increase the accuracy of your data input and help you get your work done faster!
Practice workbook for download
Multi-selection dropdown - examples (.xlsm file)
454 comments
An honestly wonderful bit of code you put together. I am curious if it is possible to limit the selection to a particular range? I have tried adding each cell of the range, but the list gets excessively long (B11:C50). Neither column nor row limitation work perfectly as it negatively impacts other parts of the worksheet.
Hopefully I am overlooking something simple. Regardless, thanks for your VBA (Very Beautiful Algorithm).
Hello! Replace the following line of code to restrict the execution of the multiple selections macro to a range of cells
If Intersect(Destination, rngDropdown) Is Nothing Then
на
If Intersect(ActiveCell, Range("B11:C50")) Is Nothing Or Intersect(Destination, rngDropdown) Is Nothing Then
I hope my advice will help you solve your task.
Hi, this is great.
I used your code for Multi-selection dropdown with item removal for a specific cell option and got it working as I wanted. However, for some reason, it broke some of the macros I had in that worksheet. All the sudden my macros started giving me "variable not defined error" in multiple instances. Deleting your code fixed the issue.
I have your code at the very top in Sheet1 followed by over 20 macros triggered by buttons
After spending some time digging for answers the problem relies in the fact that your code requires the "option explicit" line. This forces me to define every single variable in my macros.
is there any workaround other than defining every single variable in my >20 macros?
Any suggestion is welcome.
Thanks
Ok found a workaround (i think). i have to cut and paste the macros from the buttons into separate modules and then assign those modules back to the buttons.
Cheers
Hi! If the "option explicit" option appears in the module, you must explicitly declare all variables using Dim, Private, Public, ReDim, or Static instructions. You can try removing this option, but I don't guarantee the code will work correctly.
Is there a way to show the dropdown symbol in excel cell using VBA (for multi selection dropdown handled thru VBA code)?
Hi! Excel has a special method of identification for cells with a drop-down list. The code for multiple selections works in all cells with a drop-down list. Unless you specify a specific range. You could try writing VBA code to get what you want, but we don't provide that help on this blog.
Thanks for the instructions! Now I want to send this Excel file to colleagues. Tried it, and from some email addresses it bounced back. I am presuming because of the macros in the Excel file which could be seen as a security risk. I tried creating a xlsx, but it loses the multiselection functionality. Is there any way to preserve the multiselection functionality in a document format that won't be perceived as a security risk?
Hi! Unfortunately, there is no other option in Excel. You can try sending the file as an archive. Maybe this article will be helpful: How to enable and disable macros in Excel.
Brilliant work!
(One other tutorial I looked at required specifying the call with the drop-down. Ugh.)
Thank you, Alexander.
-- Jon
Thanks for this tutorial. It was very helpful.
I have created a pivot a table and I will like my multi-selections to appear individually as column headers. Can this be achieved?
Hi! Multiple selection macros only work for the drop down lists you have created.
I simply cannot discern where, within the code that appears in the "VBA code to select multiple items in dropdown list" table, that the suggested code for introducing column flexibility (below) should be inserted! Please advise. Thanks.
Multi-select drop-down for specific columns
To allow selecting multiple items in a certain column, add this code:
If Not Destination.Column = 4 Then GoTo exitError
Hi! Pay attention above to the beginning of paragraph: How to create multi-select dropdown for specific columns, rows, cells. It says where to insert this code.
Hello, thank you for this useful website.
I have questions please,
I tried making a drop down with multiple selections for my data, but when I insert the formula =INDIRECT("Table1[Items]")
I get an error message saying (the source currently evaluate to error !)
I don't why?
Another question: I cannot see from where the name "Table1" in the above formula came?
Thank you
Hi! If I understand your task correctly, this article may be helpful: Create drop-down from Excel table.
Hi,
Thanks for the information. Its really help. I'm trying to use the code in protected sheet but fail to do so.
Questions:- How im going to make the code valid for more then one table list in one sheet. Cause i tried many times but its not gonna work on your code above. Please help me regarding this. i have use these code fo yours: Multi-selection dropdown with item removal & Enable multi-selection functionality in protected sheet at my Excell. Thanks in advance.
Hi! Unfortunately, your problem is not very clear. The code works for all dropdown lists on the sheet. If you want to limit its use, I recommend you to read carefully the paragraph: Multi-select dropdown for specific columns, rows, cells. Or explain it in more detail.
Thanks for your feedback. I already follow step by step, to enable the dropdown list, that can be use and pick multiple and its work perfectly using this code: Multi-selection dropdown with item removal. Unfortunately, after I implement the code that can Enable multi-selection functionality in protected sheet at my Excell, the dropdown list can't be select as before. it only pick one instead of more than one items in one cell. if i delete this code : Enable multi-selection functionality in protected sheet at my Excell, its back to normal. Cause i need to protect the sheet at several cell and remain unprotected at several cell to user for key in the input. If you have other solution on this issues please help. Thanks in advance. Really appreciate.
added input issue:-
- I have more than one list of dropdown in one sheet. I already try add the other list of dropdown into your practice example excel and its won't work if I add the Enable multi-selection functionality in protected sheet at my Excell, So the conclusion, its fail to pick more than one items, if the sheet content more than one dropdown list in protected sheet.
A worksheet can have any number of dropdown lists with multi-selection functionality. I cannot see what you are doing. Try to carefully follow the recommendations from the article above.
Hi! The code you are given works with a protected worksheet. This method of protection is described here: How to protect Excel sheet. This protects the entire sheet, not individual cells.
There is also a lot of discussion of this problem in the comments below. For example, here.
Thanks for reply. As i understand on your comment, it is valid for the different dropdown list (one or more set of validation) or it's only valid for one list(table) of dropdown only in one worksheet. And for your information, i already go through the other comment but there is no solid answer for the issue yet.
If you look carefully, you'll read in the second paragraph of the article: "The code enables multiple selections in all drop down lists on a particular sheet. You do not need to specify the target cell or range reference in the code".
In the example file, you can see how multiple selections works for 5 dropdown lists on one sheet.
I am not an expert in excel. Your VBA code helped me a lot. But still am facing problem with the "protection".
Can u explain where to insert the protection code along with the existing code.
I did copy, paste with the existing code, but it doesn't work. do you have some pictures or videos to share.
Thank you.
Hi! You can see a sample code in this paragraph above: How to enable multi-selection functionality in protected sheet.
Hi,
I've tried to the copy paste of the code that was recommended in the paragraph above, but it didn't work. I saw other people had the some issue. Could you please give an example of the complete code including both the Multi Selection functionality as well as the code to Unprotect and protect so we could evaluate what we're not doing properly?
Or even include this type of example in the excel spreadsheet you provided as an example?
Thank you in advance
Hi!
1. Add the code you need to the worksheet. For example, Multi-select drop down without duplicates.
2. AFTER THIS CODE, add the code for working on the protected sheet from the paragraph: Enable multi-selection functionality in protected sheet.
3. That's all.
Hi,
Thanks for the code it works great. I would like to have it applied to a range of cells that are in a table like all the ones that are in "Column1" but nowhere else, how could I do that please?
Thanks in advance!
Hi! I kindly ask you to take a closer look at the following paragraph of the article above: How to create multi-select dropdown for specific columns, rows, cells.
Thanks for the article, it was very helpful.
How would I adjust the code for multi-selection dropdown with item removal to display the selected options in alphabetical order?
Ideally would want to have the same result if <1 option is selected, regardless of selection order.
Hi! To sort text in a cell alphabetically, you need a separate macro. Unfortunately, we do not plan to do this.
The multi-select code with and without removal does not work. I have read and implemented the instructions 6 times and only one item can be selected. I have saved the sheet as a macro enabled excel file. I have created the list using the table and the "INDIRECT" line of code. I have created the developer tab and added the VBA code exactly as it is shown in the article. But only one item can be selected.
You may want to add a step to your list. I had to close out of the spreadsheet entirely and re-open it for the macro to be enabled.
This code is a tremendous help. Thanks!
Hi! Unfortunately this information is not enough to understand the cause of the problem. I can't see how you added the code to your worksheet. Try using the sample file linked at the end of the article.
Worked perfectly, thanks!
So helpful!!! Thank you!
Hello,
Thanks so much for this code - it's great! One challenge I have though is that when you use the code to enable removal of previously selected items it doesn't quite work for me. Let's say I have 2 items that can be selected - QWE; RTY and am using the custom delimiter: " | "
If I select QWE, then RTY, they are now both added to the list like this : "QWE | RTY"
If I re-select RTY, it is now removed from the list like this: "QWE"
If I re-select QWE a second time, it is added to the list a second time, NOT removed, like this "QWE | QWE"
If I re-select QWE again a third time, they are both now removed and there is nothing left in the list.
The case where an item is added twice only happens when you try and remove the first item in the list. My best guess is that this because the first instance automatically doesn't have a de-limiter, so perhaps the code for removal is looking for a de-limiter, doesn't see it, then s then adds the item again with one? Then when you select a third time, it picks them both up due to the de-limiter?
I'm not sure, I'm not great with code but would appreciate if you're able to provide any other advice on this. this is the only VB I have in this document.
Thanks!
Hi! We have made changes in the code. Reinstall the code Multi-selection dropdown with item removal. Thank you for your comment!
Hi,
code was working greatly, but I have now new company notebook, suddenly code stopped working. I have downloaded your practice sheet, and it does not work as well.
No errors, dropdown list allows only one selection.
Excel version: Microsoft® Excel® for Microsoft 365 MSO (Version 2310 Build 16.0.16924.20054) 64-bit
Any suggestions ?
Hi! Maybe these articles will be helpful: How to enable and disable macros in Excel and Excel VBA macro tutorial: how to create, change, copy and delete macros.
Hi.
I understand the basics for macros to run.
OFF COURSE all macros are enabled, folders trusted.
Anyway it doesnt work. I was trying altering the code.
I deleted this section at the end and it worked for a while. Then again stopped working.
Private Sub Worksheet_SelectionChange(ByVal Target As Range)
End Sub
Looks to me like option explicit doesnt run properly.
Hi. On the old computer the code worked, on the new computer it does not work. Conclusion: your new computer software is the problem, not the macro code. Unfortunately, I am not able to solve this problem for you.
Hi Thank you for this code - it works but I wanted to take it a step further. I am using the code which places the selected items on a new line (so DelimiterType = vbCrLf), but I also want to add a comma to the previous line.
I have tried "DelimiterType = & ", " vbCrLf" - but this creates an issue because it allows an item to be selected twice, then after selecting that item a couple more times, only then do all instances get deleted.
Can you advise how to add a comma to the end of the previous line?
Thank you.
Gemma
Actually, I've just recopied the code and used "DelimiterType = "," & vbCrLf" and it seems to be working now.
Thank you
Gemma
Hi! Here's how to set the line separator correctly:
DelimiterType = "," & vbCrLf
Hi, thank you this code was very helpful. I am using the code which allows duplicates to be selected and I would like to count how many times each word is mentioned (including duplicates within cells). Do you know if there is a way to do this? I have tried the COUNTIF and SUMPRODUCT functions but cannot find a way to count the duplicates also - it counts a maximum of 1 per cell. Thanks.
Hi! See the answer in the previous comment.
Hi. Thanks for the comprehensive explanation. I have used the VBA successfully and created the multi selection list for all my worksheets - it works great! Once i have collected my data, is there any way to calculate/summarise how many of each selection within a drop down list have been selected. i.e. if i and counting fruit and have selected "apples" ten times (or 1000) and selected "pears" 37 times, how can i get excel to calculate that without me having to count each individual selection.
Hi! A drop-down list creates a text string in a cell. To count cells with specific text, try these instructions: How to count cells with certain text in Excel. I hope it’ll be helpful. If this is not what you wanted, please describe the problem in more detail.
Hi Alexander,
Thank you for the VBA. I am successfully using the code for the multi-select with removal and have added the part to do the selection on specific columns on one sheet. However, when I attempt to use the same code on other sheets in the same workbook (I have updated to point to the relevant columns in the new sheets) it does not work.
Is there something that I'm doing wrong or can this only be used on one sheet in a workbook? Any suggestions will be appreciated.
Hi! As the article above states, the code must be placed on each worksheet on which you want to make multiple selections from the drop-down list.
Hi Alexander I have your code working, but as soon as I close and reopen the file, the code won't work anymore, do you have any suggestions for me please? I check and nothing has changed, I have copied it to a new workbook and the exact same thing happens, it works until I close, then when I reopen, it will only let me select one item per cell. I did not add specified columns to the code, and my worksheet has about 10 list tables, so not sure if it's too much information? I am also very new to VB, so I could be doing something without realising I'm erring. Data tables are on a separate worksheet and the code is only on the worksheet I have the data validation on.
Hi! Note that your workbook must be saved in .xlsm format as a macro-enabled workbook. You can also find useful information in this article: How to use and store custom functions in Excel
Hi - thanks for this code - it works really well, except (as many have said) when I try to add the code for a protected sheet.
Could you perhaps show EXACTLY where this code is meant to go?
Should it be split and replace areas of the primary code? Tagged on at the end? It really isn't clear how to make this work.
Many thanks
Hi! Pay attention to the following paragraph of the article above: How to enable multi-selection functionality in protected sheet. No changes need to be made to the primary code.
Hi and thanks for the prompt reply. I still can't figure it out though and have tried it on both the sample sheet and my own, using office 365 Excel
I have appended the extra code following the primary code and I have set a password in the extra code. This is what happens:
I set the multi picker cells to be editable when the sheet is locked. At this point the sheet is unlocked.
I select an item in one of the multi picker cells. The sheet is then locked by the code.
This cell is then reverted to a single option pick list but is editable.
If I step on to another multi picker cell, the sheet unlocks.
As soon as I make a selection in this cell, the sheet is locked again.
None of the cells allow multi selection when the sheet is protected.
I know you have responded to this on several occasions but it really isn't clear where this extra code actually goes, to make this work or why it isn't dong for so many.
Many thanks
Hi! I think you understand that I can't check the code in your workbook. We have already discussed a similar problem in the comments above. I cannot reproduce your issue. Try deleting all the code and installing it again. I hope this will help.
Hi!
Thanks for sharing this. I tried the code, and it works for examples given above.
However, I would like to use the multiple selected items as input for a function (e.g. FILTER function).
Let's say I have a dataset for several years, and want to do a report with the filter function, filtering data for selected years, but giving the user the ability to select one or more years from a dropdown list.
Is there a way to do this?
Thank you in advance for your help. :)
Hi! The macro allows you to create a text string of multiple items in a single cell. But I cannot advise you how to use this text in the FILTER function. Each criterion of the FILTER function must be written separately.
After selection of multiple items, I want to add the input data. what should I do?
I am not sure I fully understand what you mean.
Hi - thanks for the code. When I use this I get the multi-select list working fine - however Excel is showing the standard 'data validation' error on the cell, as a concatenated version of selections isn't valid according to the original list defined in the data validation tab.
Is there any way to get Excel to not show up the issue?
Further, I cant use the formula bar to edit the result (i.e. manually delete an entry selected), because again, it's not valid based on the original formula list provided.
Any help would be appreciated!
Also, the password protection seems to be a bit funny! The code seems to turn protection on before I have, and then the list doesn't work anymore?
I fixed the first by protecting the sheet - and the second I used different code.
Sub Example()
ActiveSheet.Unprotect
Program logic...
ActiveSheet.Protect
End Sub
Around the sub I copied. Thanks :)
Hi! Sorry, I do not fully understand the task. Please clarify your specific problem or provide additional information to understand what you need. I can only say that the macro does not affect the work of the dropdown list in any way.
first of all thank you very much, The code is really good and works great ! 😊
I saw that people wrote that they have a problem when the sheet is protected. I also had a problem exactly like this, that the multipicklist worked fine, but as soon as I make the sheet protected, the multipicklist becomes a normal picklist. And even when I added your code for the protect it didn't help. I investigated your sample file which continues to work fine even when the sheet is protected, And I tried to understand what is the difference between us. I think that I came to a conclusion as to what is causing the problem:
When a different cell with a different validation is defined in the sheet, it prevents the multipicklist from working when the sheet is protected. I you will add to your sheet another validation in other cell, you will probably see the problem.
I really don't understand VBA, I would appreciate if you have an idea what can be changed in the code so that it works even when there are several different validations in the sheet.
Thank you very much !!
Hi! If I understood the problem correctly, you can only use one macro per worksheet. It is written about it in the article. All macro variants are in the article. You can add the code for the protected worksheet to any macro, as described above. I hope I have answered your question.
Thanks for the replay.
I m using only one macro on the sheet.
But I have two cells with different validations. Only one of them needs to be a multipicklist and uses your macro, the other validation is a number validation and doesn't have a list at all.
When I protect the sheet and add your code for protecting, the multipicklist becomes a regular picklist. It doesn't run the code correctly.
I tried to debug (As I said before, I don't know VBA, but I am programming in other languages). when I select a value from the list, I see that on the line "If rngDropdown Is Nothing Then GoTo exitError " it falls and goes to exitError without doing all the other important lines.
I would appreciate your help please. Thanks.
Hi! I downloaded the sample file at the end of the article and added the code for the protected sheet. I did not get any errors. Try reinstalling the macro code.
Your code works great, I didn't say it didn't.
But I meant that if you add another validation in another cell (like a validation on a number) and then you will see the problem.
In any case, my problem was solved in another way, the client asked to remove his field from the file 😀.
As I said, I added another validation in another cell (validation on a number) but I didn't see any problems. There is some problem in your file and maybe reinstalling the code would solve it.
Hi,
Amazing work. I have successfully implemented the code. However, when I transfer the file through e-mail, and then download it again, the multi-selection dropdown doesn't seem to work any more. Would you happen to know a solution to this problem?
I have resolved this issue, it was due to the excel being a read-only file. I saved it as another file and it worked.
Thanks again.
Hi,
Further to my previous question, how can I apply this code to only certain columns, as I have some columns that should only allow a single selection, where there are three columns ranges("A3:A5000, P3:P5000, AL3:AL5000") which should allow multi-select?
Please re-check the article above since it covers your task.
Hi,
Thanks for the great share.
I have multi-select dropdowns in a number of columns which works as per your post. However, if this is applied to column A the macro does not seem to work as it does for all other columns. I have tested the dropdown without the VBA and it works for single selections. All dropdowns are setup exactly the same, just pointing to different sources. Any idea what might be causing this?
Hi! The code works for the dropdown list in column A just as it works for the other columns. You may have set a limitation for some columns, as described in the article above.
Thank you for this code. It has been really helpful. If I want to be able to scroll through the drop down menu rather than using the arrow buttons to go through the list, how would I do that?
Hi! This code allows a standard Excel drop-down list to write multiple values into a cell. Everything you can do with the drop-down list is described in this article: How to edit, copy and delete drop down list in Excel.
Hi there! This is a fantastic feature but I’m having a hard time thinking through the best way to create a pivot table based on the individual components. For example, I don’t want to know the count of “Bread, Eggs, Butter” I want to know how many times Eggs are on the list and for what dish. I know I can do =countif(range, “*”&”Eggs”&”*”) for the count but I’m not able to do that in a pivot table to do additional analytics. I appreciate any guidance you’re able to provide! Thanks!
Hi! I don't really understand what your question has to do with the article above. If you want to count how many times a specific word occurs in a range of text cells, use the SUMPRODUCT function and these guidelines: How to find substring in Excel. For example,
=SUMPRODUCT(--ISNUMBER(SEARCH("eggs",B1:B10)))
In a pivot table, you can't get any analytics on a part of a text string. You need to split the text into individual cells.
This worked perfectly when I first tried it out, many thanks! However, when I got an update of MS Office, the code does not work for 64 bit - any way this could be converted easily?
Thanks!
Hi! The drop down list with multiple selections macro works in any desktop version of Excel that runs VBA. Try reinstalling the macro. You may find this article helpful: How to enable and disable macros in Excel.
I'll try, thanks!
Thank you this is perfect!!
Thank you for your help! I inserted the primary code into my spreadsheet and it worked as described. However, when I tried to add in the additional code to enable a multi-select dropdown functionality in a protected worksheet, I would get the following error: Compile error: Ambiguous name detected: Worksheet_SelectionChange
It would specifically highlight the first line of the added code which followed the primary code. I tried both protecting the sheet and workbook individually and together, and each time I would get the error. The last group in the primary code has the same "Private Sub Worksheet_SelectionChange (ByVal Target As Range)" language. I tried replacing that with the additional code, but that only resulted in the multi-select dropdown no longer functional. I have updated the password options as instructed.
I don't see this in the practice workbook, and I'm not sure how to fix. Any help you can provide would be much appreciated. Thanks!
Hi! Check your code carefully. Private Sub Worksheet_Change occurs there twice. Delete the unnecessary code.
Sorry, the primary code I used was for the "Multi-selection dropdown with item removal" option. So that only has one occurrence of "Private Sub Worksheet_Change". The ending version is "Private Sub Worksheet_SelectionChange". I combined this version of the primary code with the "multi-select dropdown functionality in a protected worksheet", leaving 2 occurrences of "Private Sub Worksheet_SelectionChange".
I am trying to create a shared document for work using the Multi-selection dropdown with item removal and it works great however it doesn't cross over to the online version (which all my colleagues would see).
It still lets me select from the list, the most basic form of it works, but the mutli selection and item removal doesn't seem to be translating over.
Any idea how I can fix this?
Hi! See answer in this comment.
Hi, thanks for the code. Selecting multiple options works smoothly, but if by mistake I select a wrong item in the list, how can I remove it? nothing seems todo the trick. thanks in advance
my bad, apologies, I used the wrong code snippet. all works perfectly
Hi! To remove the wrong item from a cell, you can use the code: Multi-selection dropdown with item removal. Or you can manually correct the data in the cell because the dropdown list creates a text string. You can edit it in the formula bar.
Hello,
This code works beautifully, until I upload my spreadsheet to onedrive so that I can share it with colleagues, it then loses the option to select multiple options from the drop down list and only allows you to choose one, Is there a way to change the VBA code for a share point excel spreadsheet, Or is there another way to add a multiple selection list for one that opens on chrome ? Any help would be appreciated
Hi! Remember that VBA only works in the desktop version of Excel. VBA macros and functions do not work in Excel Online.
Hello,
This is all great information!! So far is working perfectly! I do have a question, Is there any code that could eliminate your selection from the drop down box in the next row? For example, If I have multiple people using the excel spreadsheet and we have 2 rows with employee names in the drop down list, 1 person picks 3 names from the list and the next person in the next row needs to see what names they cannot pick.
Hi! You can try writing code that will dynamically change the data to create a dropdown list.
Thank you very much for providing this code, it works just how it's suppost to! I used the dropdown with multiple selections in separate lines code and realized, that the code does not keep the entries in the order of the drop-down menu. For instance: If you select Apricot and then Bread crumps, it will show it in the right sequence.If you de-select Apricot, it correctly only shows Bread crumps. However if you then select Apricot again, the result will be displayed at Bread crumps, Apricot. Is there a way to keep the selections in the order of the drop-down?
Hello! Since the drop-down list creates a text string in the cell, you need to write special VBA code to sort the words in the text string within the cell.
I am having a problem getting the code to work that allows the multi select in a protected workbook. Any suggestions? I’m not getting a specific error, the multi select functionality still doesn’t work when the additional code is added and it is updated with my password. Thanks!
Hi! Unfortunately, this information is not enough to understand your task. Carefully follow the recommendations from the article above. You can use the sample file. See the link at the end of the article. Or explain your problem in detail. What do you want to do and what code are you using.
The drop down list with multi- selection is great.
Is there any code that could eliminate your selection from the drop down box and repopulate it in the next cell over when it is clicked?
Hi! Such a code does not currently exist, and there are no plans to create one.
Hello,
Thanks so much for these codes. It works perfectly. Would it be possible to enhance the code to provide user with long description in the drop down list, but returns the short abbreviation in the worksheet? For example, when entering a state, user types in "A" and the drop down shows "Alaska, Alabama, Arizona, Arkansas". If user selects "Alaska", the worksheet would record "AL".
Best Regards.
Hi again,
please ignore. I figured out how to accomplish what I needed. In the line "newValue = Destination.Value", I simply replaced it with "newValue = Left(Destination.Value, InStr(1, Destination.Value, "-") - 1)". Of course, the state list would have to be entered as "AK-Alaska"; "AL-Alabama", ... Drop down list would show "AL-Alabama", but when user selects it, worksheet will show "AL".
Many many thanks for the codes.
Your request goes beyond the advice we provide on this blog. We don't do customization of the code.
Hi
Thanks for the code. It works perfectly and it saved a lot of our time.
Just wondering if it is possible to have different text colour for each phrase in one cell.
Let say in your example, whenever 'Milk' is selected, it shows with a red text colour and the rest item are shown black. Is this something possible?
BTW, I already tried the cell rule but it will change the text colour for entire cell not one item.
Many thanks
Hi! You can have a different text color for each phrase in one cell using a VBA macro. It is impossible to do this using regular Excel tools. Here is an example of such a custom function: How to highlight duplicate text strings or words in Excel cell.
First, thank you for sharing this code. I'm excited to see it in use with my current worksheet. However, I have a question first: where do we insert this code? You wrote, "insert the VBA code at the back end of the target worksheet" without details for what that means.
Hi! Read the entire article. Instructions are provided below in this paragraph: Insert VBA code to allow multiple selections.
Hi, thanks for our tutorial!
May I know if I can filter those "multiple selections" data that we just created?
Hi! The drop-down list with multiple selections creates a text string. To create a filter on a part of this text string, you can try using the advanced filter options as described in these instructions: Filter text data.
I have just downloaded your file and I dont have any error. Your example is working perfectly on every tab without error.
I just don't get why yours is perfect but then your code injected in ours won"t behave exactly the same way.
PS : This should be the last post, apologies for multiple posts for the same topic.
Hi,
Forgot to mention the nature of the error. It is a data validation error. It behaves as if the multiple options selected could not match when any of the options defined in the drop down list. In a way, it is true if the validation compares the value of the cell built with multiple entries with a cell defining the drop down list. In this case, there can't be a match hence we get an error flag.
Surprisingly, looking at your example, it seems you don't meet the same behaviour.
Best regards,
Hi,
Thank you for this valuable multiselection piece of VBA. Unfortunately, I have the same issue with an error flag on each cell now. The multi selection is working and even with your code enabled, there is no error flag if you select one option only. The error only comes when you select at least 2 options from the drop down list.
I can't appy BThompson's suggestion as I only have 1 table.
Any other suggestion please?
Best regards,
PS : I guess the filtering on this column with multiple options selected will not allow filtering by one of these options only, right? Any suggestion to allow "smart" filtering once multiple options are entered in a single cell?
Hi! You said earlier that the sample file you downloaded works without errors. So, the problem is in your file. Check what VBA codes are in your file. Maybe some other code is preventing the macro from working. You may have characters in your data that you also use as delimiters. We discussed this in the comments above. I can't check your file.
The drop-down list creates a text string. To create a filter on a part of a text string, you can try using the advanced filter options as described in this guide: Filter text data.
Hello, I used your script and it works. However, the macros won't run for excel online. Do you have any idea how to fix it?
Hi! I already answered in the comments above that Excel Online does not work with VBA. Therefore, macros and user-defined functions do not work there.
This is great! One issue, I am now getting a data validation error flag on every cell where I have selected multiple items from the list. The field type information is - Data type: List Restriction: Value must match one of the listed items. Blanks are ignored and are automatically valid.
I tried unchecking the 'show error alert after invalid data is entered' box in data validation but the error flags are still appearing.
Hi! Unfortunately, I don't see your data and the cause of your problem. Check if the dropdown list works without the code. Then carefully copy the code to your worksheet. Or use the example file linked at the end of the article.
I ran into the same error. Try spacing your tables apart at least one column. Otherwise, your individual tables will be treated as part of the larger, original table.
I have the same data validation issue. It seems isolated to when your data is in a table instead of just a collection of columns