3 ways to find all combinations from a set of numbers that sum to a specific total.
Finding combinations of values that add up to a given sum is a common problem in data analysis. For example, you may want to know all possible combinations of items that can be purchased with a given budget, or all possible ways to allocate resources to meet certain requirements. In this article, we will explore how to use Excel Solver and VBA to accomplish the task. We will also discuss some potential pitfalls and limitations of each approach that may arise when handling large datasets or complex constraints.
Find a combination of numbers equal to a given sum with Excel Solver
Regrettably, none of inbuilt Excel functions can help you identify the numbers that add up to a given total. Luckily, Excel provides a special add-in for solving linear programming problems. The Solver add-in is included with all versions of Excel but is not enabled by default. If you are not familiar with this tool, here's a great article on how to add and use Solver in Excel.
With the Solver add-in activated in your Excel, proceed with the following steps:
- Create the model.
For this, enter your set of numbers in one column (A3:A12 in our case) and add a blank column to the right of your numbers for the results (B3:B12). In a separate cell (B13), enter a SUMPRODUCT formula similar to this:
=SUMPRODUCT(A3:A12, B3:B12)
- Run the Solver add-in.
On the Data tab, in the Analysis group, click the Solver button.
- Define the problem for Solver.
In the Solver Parameters dialog box, configure the objective and variable cells:
- In the Set Objective box, enter the address of the formula cell (B13).
- In the To section, select Value Of and type the desired sum value (50 in this example).
- In the By Changing Variable Cells box, select the range to be populated with the results (B3:B12).
- Add the constraints.
To specify the constraints, i.e. the restrictions or conditions that must be met, click the Add button. In the Add Constraint dialog window, select the resulting range (B3:B12) and pick bin from the drop-down list. The Constraint will be automatically set to binary. When done, click OK.
- Solve the problem.
When taken back to the Solver Parameter dialog window, review your settings and click the Solve button.
A few seconds (or minutes) later, the Solver Results dialog box will appear. If successful, select the Keep Solver Solution option, and click OK to exit the dialog.
As a result, you will have 1 inserted next to the numbers that add up to the specified sum. Not a user-friendly solution, but it's the best that out of the box Excel can do.
For visualization purposes, I've highlighted the cells that give the desired sum in light green:
Limitation: Excel Solver can find, at most, just one combination of numbers that equals a specific sum.
Find all combinations that equal a given sum with custom function
To get all possible combinations from a given set of numbers that add up to a certain value, you can use the custom function below. If you are new to UDFs, you'll find a lot of useful information in this guide: How to create custom user defined functions in Excel.
How this function works
The main function, FindSumCombinations, calls a few subsidiary functions that implement smaller sub-tasks. The function named SumUpRecursiveCombinations executes the core algorithm that finds all possible sums in the specified range and filters those that reach the target. The ArrayToString function controls the form of output strings. Three more functions (SumArray, AddToArray, and CopyArray) are responsible for processing intermediate arrays: each time we create a temporary array, add one element from the source array to it, and check if the target sum is reached. The core algorithm is taken from this Stackoverflow thread, thank you guys for sharing!
Syntax
From the user's perspective, the syntax of our custom function is as simple as this:
Where:
- Range is the range of numbers to test.
- Sum is the target sum.
Note! The custom function only works in Dynamic Array Excel 365 and 2021.
How to use the FindSumCombinations function:
- Insert the above code into a Code module of your workbook and save it as a macro-enabled workbook (.xlsm). The step-by-step instructions are here.
- In any blank cell, enter a FindSumCombinations formula and press the Enter key. Make sure there are enough empty cells to the right to output all combinations, otherwise the formula will return a #SPILL error.
For example, to find all possible combinations of numbers in the range A6:A15 that equal the sum in A3, the formula is:
=FindSumCombinations(A6:A15, A3)
As with any other dynamic array function, you enter the formula is just one cell (C6 in the image below) and it populates the results into as many cells as necessary. By default, the comma-separated strings are output in a row:
To return the results in a column, wrap the custom function into TRANSPOSE like this:
=TRANSPOSE(FindSumCombinations(A6:A15, A3))
To output the strings in the form of an array enclosed in curly braces, modify the ArrayToString function as follows:
The results will look similar to this:
Limitation: This custom function works only in Dynamic Array Excel 365 and Excel 2021.
Get all combinations that equal a given sum with VBA macro
The custom function described above returns the combinations of numbers as strings. If you'd rather have each number in a separate cell, this macro will be helpful. The code is written by another Excel expert Alexander Trifuntov who has been actively helping users to solve various Excel problems on this blog.
Start by adding the following code to your workbook. For the detailed steps, please see How to insert VBA code in Excel.
Next, create a UserForm with the following design and properties:
After completing the form's design, add the code for the form. For this, right-click on the form in the Project Explorer and select View Code:
Tip. Instead of re-creating the form from scratch, you can download our sample workbook at the end of this post and copy the Code module and UserForm1 from there (see how to copy a macro to another workbook). To make the macro accessible in all your workbooks, copy it to the Personal Macro Workbook.
With the code and form in place, press Alt + F8 and run the FindAllCombinations macro:
On the form that pops up, define the following:
- The range with the source numbers (A4:A13)
- The target sum (50)
- The upper-left cell of the destination range (C4).
When specifying the upper-left cell of the output range, make sure there is at least one blank row above (for headers) and enough blank cells down and to the right. If there are insufficient blank cells, your existing data will be overwritten.
Clicking OK will produce the result like shown in the screenshot below:
- In C4:C6, you have the combinations of numbers as comma-separated values.
- Columns F, G and H hold the same combinations of numbers, with each number residing in a separate cell.
- In D4, you have the target sum.
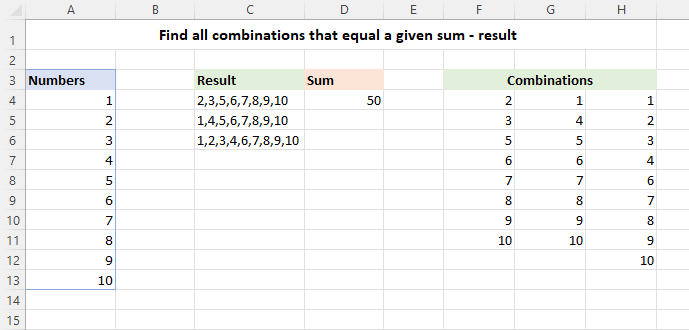
This form of output makes it easier to check the result - just enter the SUM formula in cell F13, drag it to the right across two more cells and you will see that each combination of numbers adds up to the specified value (50).
Limitation: For a large set of numbers, the macro may take some time to generate all possible combinations.
Advantages: Works in all versions of Excel 2010 - 365; provides two forms of output - strings of comma separated values and numbers in separate cells.
In conclusion, finding all combinations of values that equal a certain value is a powerful tool for solving a wide range of data analysis problems. Hopefully, this article has provided you with a useful starting point for exploring this topic further and handling similar problems in your own work. Thank you for reading!
Practice workbook
Find combinations that equals a given sum - examples (.xlsm file)
108 comments
Hi,
This is a great solution and works well - I wondered if you could help me with a query though. instead of returning the number values is it possible to return the values one column to the left (which in my case are text descriptions?)
Thanks! :)
Hello Paul!
You can get the numbers, and then extract their corresponding text values from another column using INDEX MATCH formula.
Hi,
Thanks for the response - I've been using the custom function and my dataset has some duplicate numbers (exactly the same) and also they are monetary figures so have a 2 decimal places.. so not sure INDEX MATCH would provide what I need.
I've noticed that some of the results aren't accurate to 2 DP.. i think there must be some rounding or tolerance in how it provides the results? - not sure how to improve this - a neat solution I just wished it was a little more robust/accurate
Hello Paul!
To avoid rounding problems in Excel, I recommend using the integer numbers. If your numbers have two decimal places, you can multiply them by 100, do a calculation, and then divide the chosen numbers by 100.